First lines of C
In the post, you will be learning to write your first lines of C code, and how C code interacts with your machine
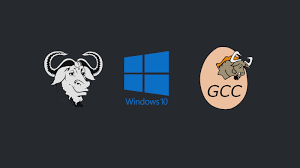
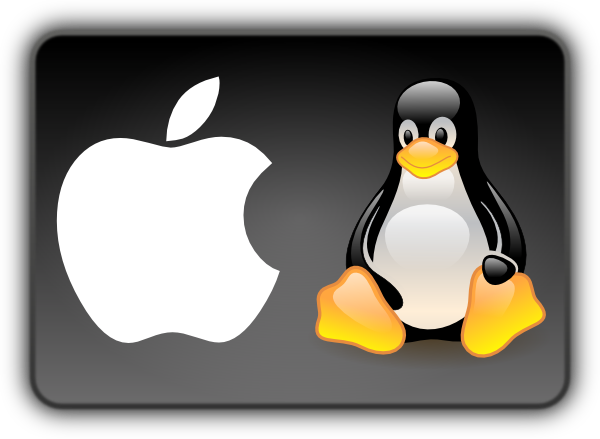
For Mac and Linux users, there is a built-in C compiler called make, so users can just use Visual Studio Code's built in terminal to use the preinstalled compiler, called make.
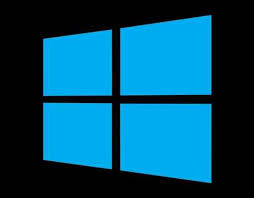
For Windows users, head to https://www.mingw-w64.org/ to install GCC. The process is quite complicated, and detailed instructions to install the compiler for Visual Studio Code can be found here: https://code.visualstudio.com/docs/cpp/config-mingw.
Once we have completed setting up our code editor and compiler, we can start writing our first C file. To start, we have to create a C file. I will be doing this with Visual Studio Code, but the process is similar for other code editors. First, open Visual Studio Code, and click on the “File” tab on the top left corner of the screen. We then click on “New File”, and a new tab will open up. We then save the file by pressing file, save as, and then typing in the name of the file, with a .c extension. For this example, I will be naming the file “hello.c”.
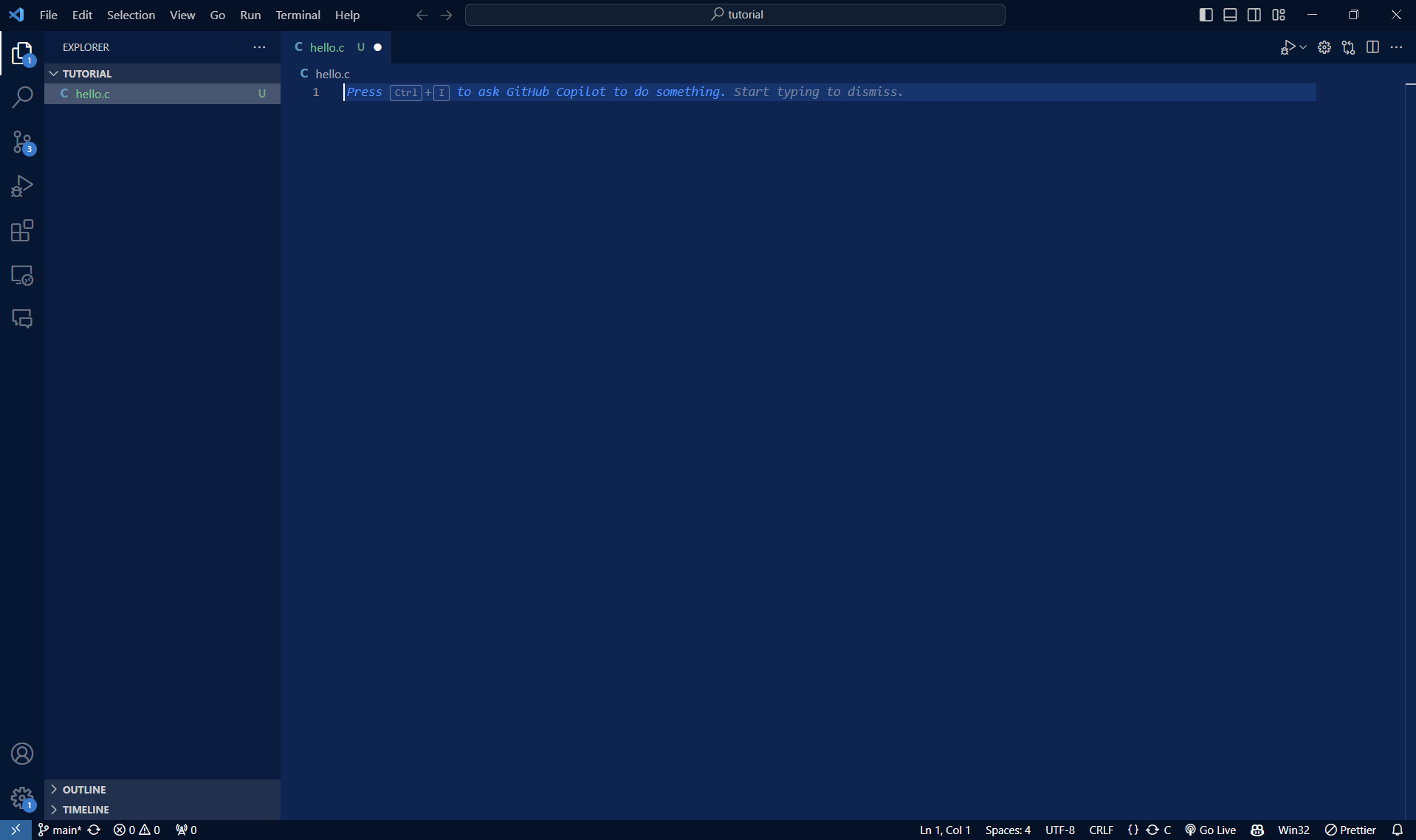
To use C to print out a string (a sequence or "string" of characters) we must first include the stdio header file, as the printf function exists in the header file. A header file is a file with functions that have already been written, and we can use them in our code. The previous blog has talked about the compilation of C code. The contents of header files are included to our code by the preprocessor, allowing us to use the functions in the header file. stdio is a shortened version of standard input/output. It usually comes pre-installed with C compilers, so we don’t have to worry about installing it. Standard input output generally means being able to take input from the keyboard and outputting to the screen. To include the header file, include this line of code at the top of your file:
#include <stdio.h>
#include is an instruction for the preprocessor, telling it that we must include the contents of the specified file into our code. <stdio.h> is the name of the file that we are including.
Next, we will write the main function. The main function is where code first starts running, and all C programs must have a main function. Inside the main function, we will write the code that we want to be run. To write the main function, we write this code:
int main(){
// Code we want to run:
return 0;
}
To start, the "int" part of the code means that the main function will be returning an integer value. This means that the
function should spit us a number when it is completed running. Next, main is the name of the function we are running. The normal brackets next to
are indicating that the function is not taking arguments, meaning that the main function does not need any external input to run. The curly braces
encapsulate what we would like to run inside the function. The "//" is a comment, and anything after the "//" is ignored by the machine when it is compiling.
Comments help developers understand and read code. The "return 0;" line is the last line of the function, and it tells the function to return the number 0 when it is done running.
This line is not explicitly necessary however, as the function will return 0 by default when it is done running. The next post will go more in depth about integers and strings (data types).
Now that we have initialised the main function, we can write the code that we want to run. To print a string, we use the printf function. This function outputs characters to the screen. Now to type Hello World!, we write
printf("Hello World!");
The semicolon at the end of this line of code is necessary, as it signals to the compiler that this instruction is complete. The double apostrophes are to signal the string we want to print.
Now you have learnt how to write your very first lines C code! Practice by writing the code to print Hello World! below!
Now to actually run this code, save the file with the written code in it. We now have to run this code through the compiler, so that we can run the code. If you are on Visual Studio code, you can install the C/C++ extension and Code Runner extension which will allow you to compile and run the code. On other code editors, you will have to compile the code through the command line. This is done by opening the terminal and then directing the terminal to where we want to compile the code. The Windows command "cd" changes the working directory, meaning that the terminal will be looking at the folder that we want to compile the code in. To compile the code, we type "gcc c –o [program_name].exe [program_name].c". The "-o" flag is used to specify the name of the output file. After compiling the code, we can type the name of the output file that we have specified to run the code. For example, since my file is called hello.c, I will type out "gcc c -o hello.exe hello.c".