Arrays in C
Learn what arrays are in the C programming language.

Arrays in C allow developers to store multiple variables in one place. This is useful when you would like to group together a list of variables to use for later. Arrays are declared by specifying the type of the array, the name of the array, and the size of the array. For example, to declare an array, we use the following syntax:
#include <stdio.h>
int main(){
int array1[5];
array1[0] = 1;
array1[1] = 2;
}
Here, we declared an array of 5 spaces. It will be storing integers, as declared and is named array1. Following, in spaces 1 and 2 we have placed the integers 1 and 2. However, a more observant reader may notice that the space in where we placed the integer 1, we wrote array1[0]. This is because arrays in C start from zero, meaning that the first space in the array is index 0. Index is the term used to describe the position of a variable in an array. We had also declared that the array will be 5 spaces, however if we would like to have an unspecified amount of spaces, we can put nothing in the square brackets where we declared the array. This is called a dynamic array, and we can specify the size of the array later in the code.
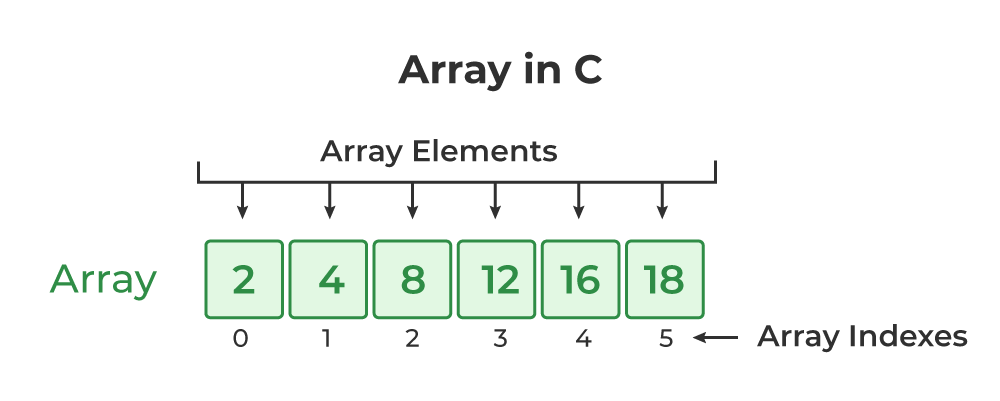
Furthermore, there are other ways to add elements to arrays by using the following syntax:
#include <stdio.h>
int main(){
int array1[5] = {1, 2, 3, 4, 5};
int array2[]; //This is a dynamic array
for (int i = 0; i < 5; i++){
array2[i] = i;
}
}
To start, we have assigned 5 elements to array1 right as we declare it. This allows elements to efficiently be assigned as soon as they are declared. Next, array2 is declared as a dynamic array, and then a for loop (check last blog for more information on for loops) goes through and assigns four elements to the array.